Wallet Integration
Connect and interact with Solana wallets in the Gold Digger ecosystem
Overview
Gold Digger provides seamless integration with popular Solana wallets, allowing users to connect their wallets, sign transactions, and interact with the Solana blockchain. This integration is essential for minting NFTs, participating in staking, and accessing the marketplace.
Multiple Wallet Support
Connect with Phantom, Solflare, and other Solana wallets
Secure Authentication
Sign messages to verify wallet ownership
Transaction Signing
Sign and send transactions directly from the dashboard
Supported Wallets
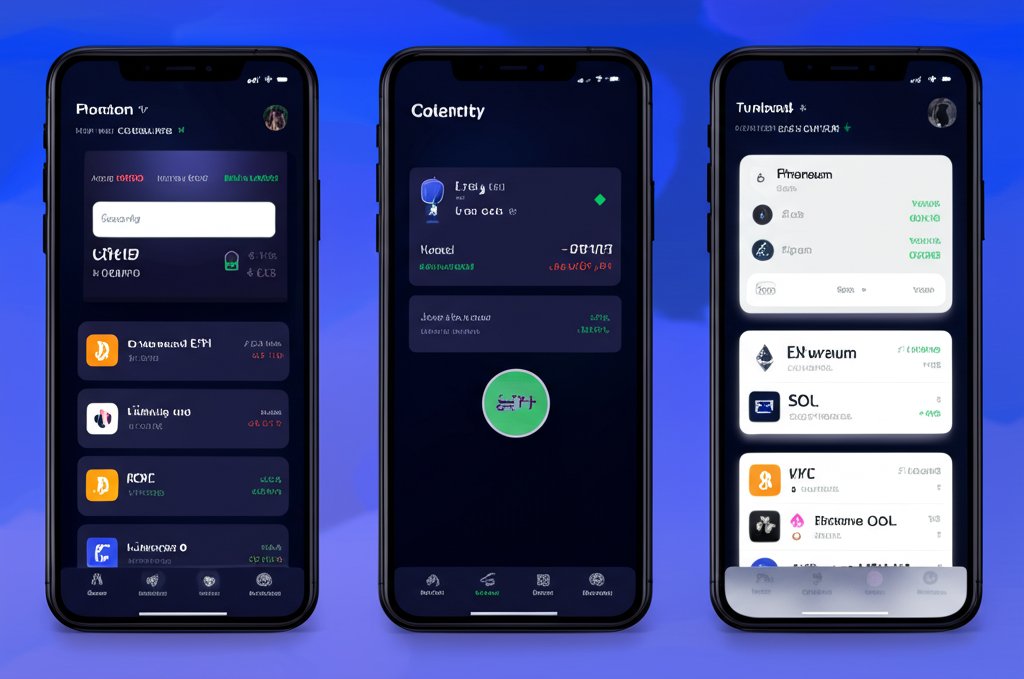
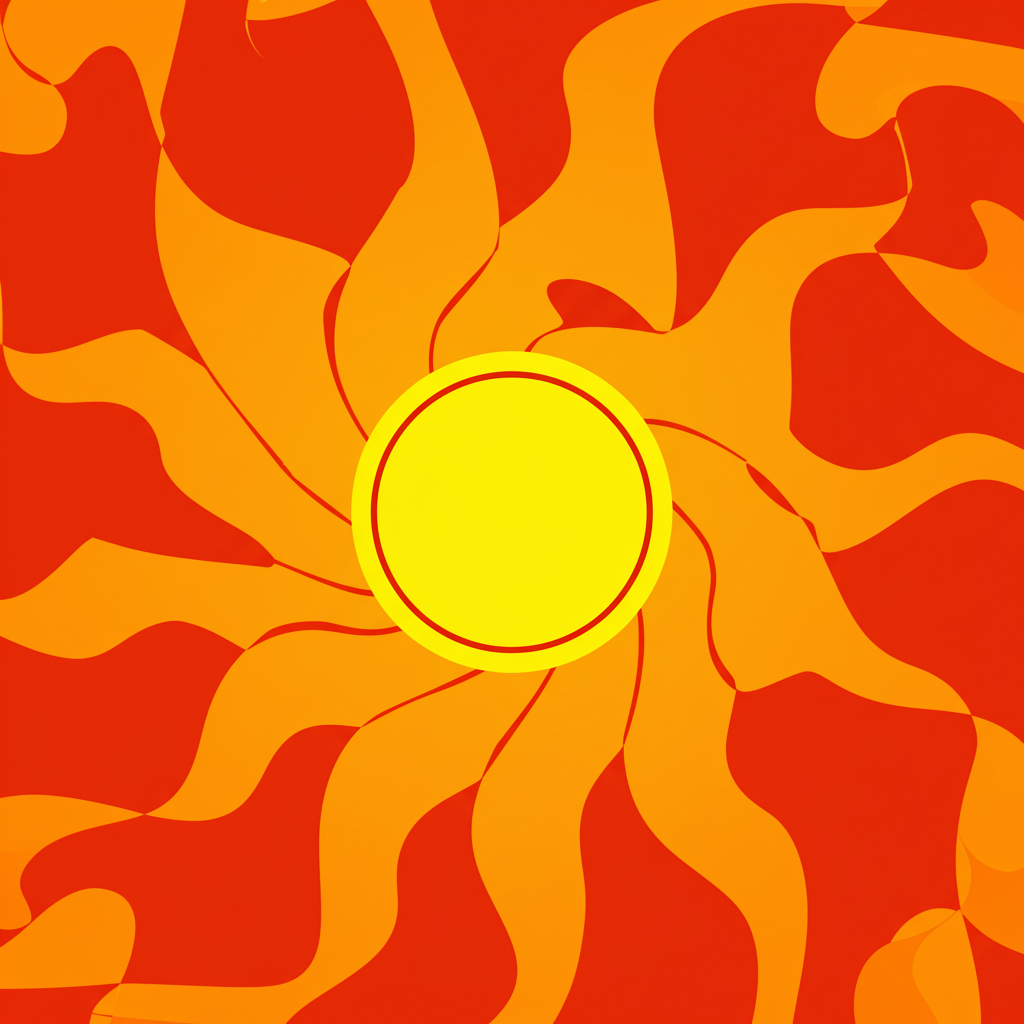
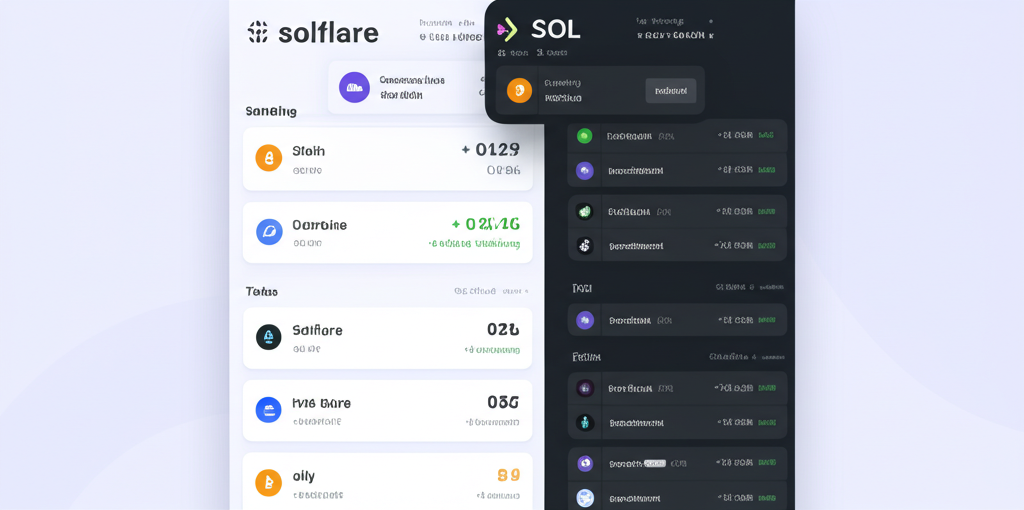
Integration Process
Gold Digger uses the Solana Wallet Adapter library to provide a consistent interface for connecting to different Solana wallets. The connection process is handled through our WalletProvider component.
// Example of connecting to a wallet import { useWallet } from '@solana/wallet-adapter-react'; function ConnectButton() { const { wallet, connect, connecting, connected } = useWallet(); return ( <button onClick={connect} disabled={connecting || connected} className="px-4 py-2 bg-gold text-black rounded-md" > {connecting ? 'Connecting...' : connected ? 'Connected' : 'Connect Wallet'} </button> ); }
Important
Best Practices
Always Handle Connection Errors
Implement proper error handling for wallet connection failures. Provide clear feedback to users when connection attempts fail.
Respect Wallet Permissions
Only request transaction signing when necessary. Always explain to users what they are signing and why.
Implement Auto-Reconnect
Save the user's preferred wallet and attempt to reconnect automatically when they return to your application.
Provide Network Selection
Allow users to switch between Mainnet, Testnet, and Devnet for testing purposes.
Monitor Network Status
Check for network congestion and adjust transaction priorities accordingly. Implement retry mechanisms for failed transactions.
Troubleshooting
Wallet Not Connecting
- Ensure the wallet extension is installed and unlocked
- Check if you're on a supported browser
- Try refreshing the page
- Verify that you're not in private/incognito mode
Transaction Failures
- Check if you have sufficient SOL for transaction fees
- Verify that you're connected to the correct network
- Ensure transaction parameters are correct
- Check Solana network status for congestion issues
Authentication Issues
- Make sure you're signing the correct message
- Check if your wallet address matches the expected address
- Try clearing browser cache and cookies
- Ensure your wallet is properly unlocked