Program Architecture
A comprehensive overview of the Gold Digger Factory Program architecture, components, and integration points.
The Gold Digger Factory Program is built on Solana's programming model, utilizing the Anchor framework for development. The architecture follows a modular design that separates concerns while maintaining efficient interaction between components.
This architecture enables the program to handle complex operations like NFT minting, staking, and token transfers while maintaining security, scalability, and extensibility.
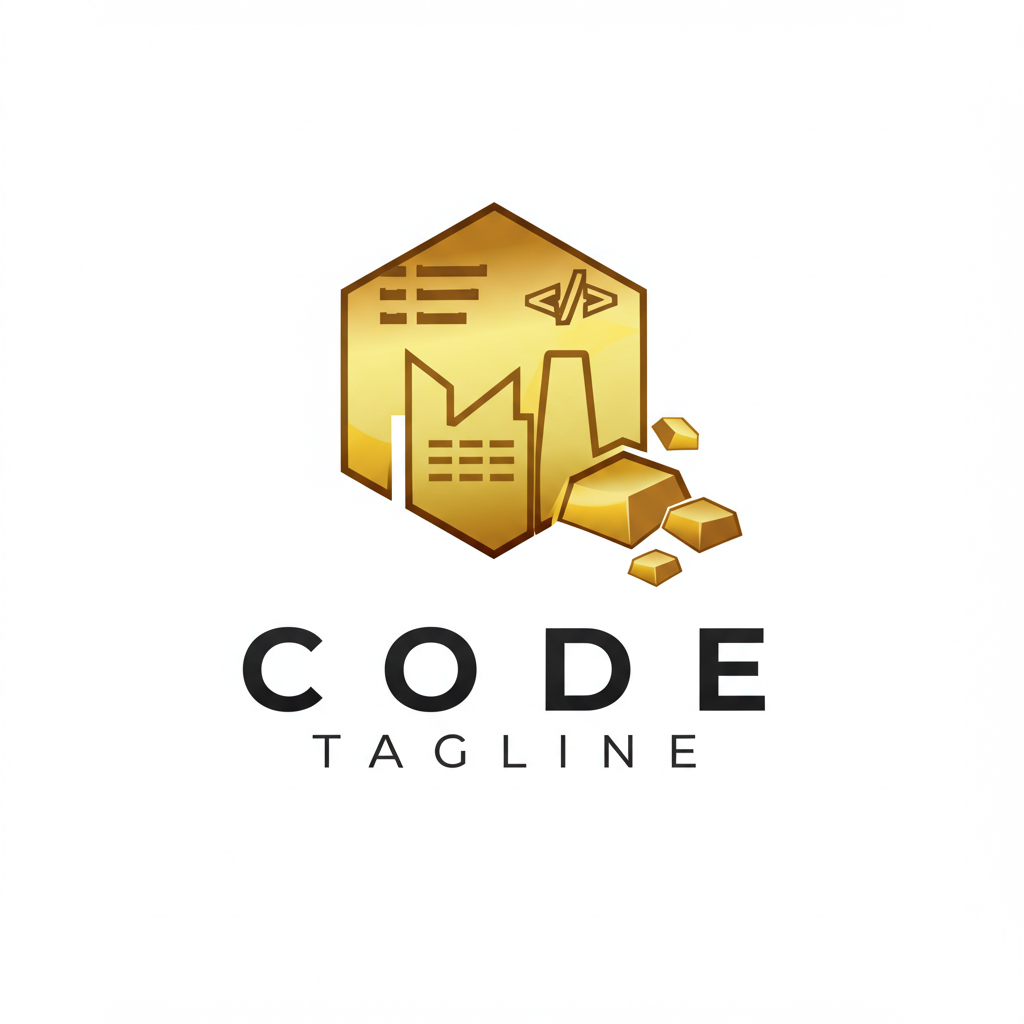
Gold Digger Factory Program
System Architecture Diagram
This diagram illustrates the key components of the Gold Digger Factory Program and their interactions with external systems. The central Factory Program coordinates with Solana's native programs, Metaplex, and price oracles to deliver its functionality.
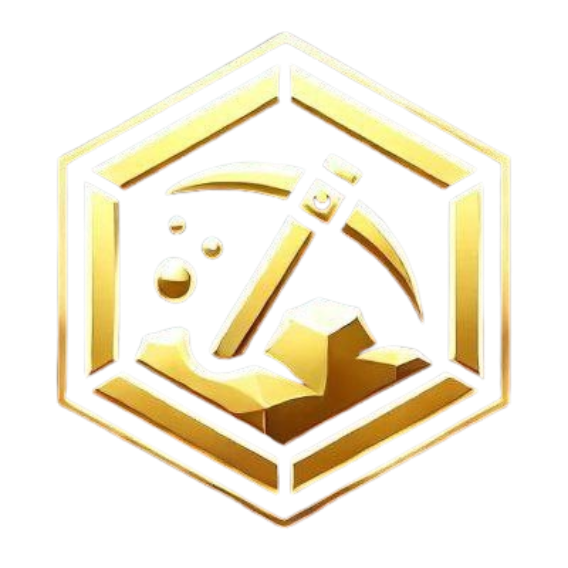
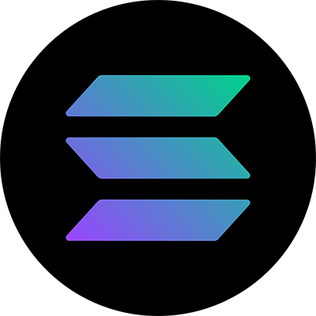
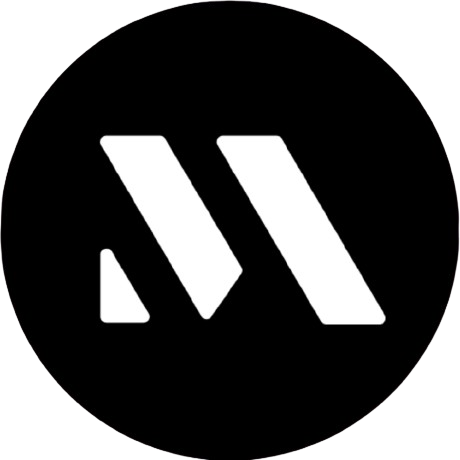
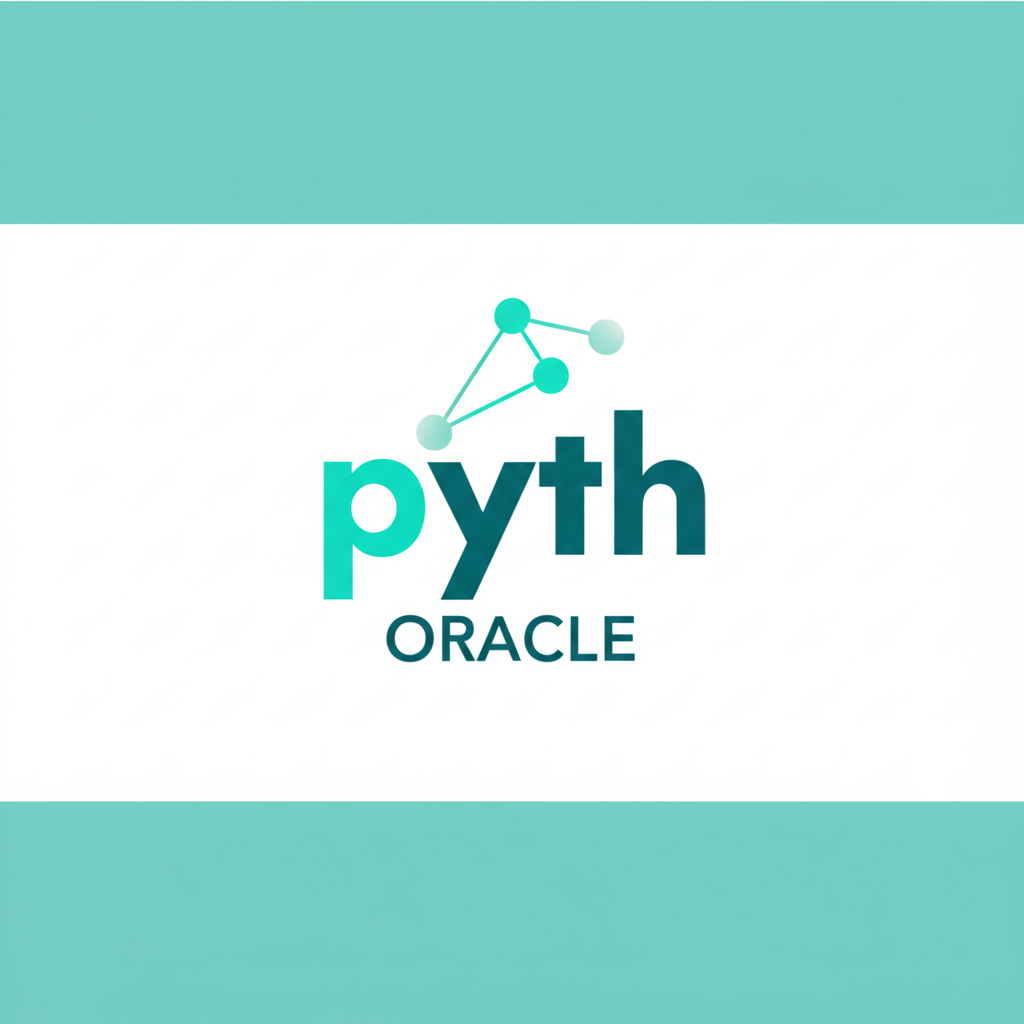
Note: Hover over components to see their relationships. The Gold Digger Factory Program sits at the center, coordinating all operations.
Core Layer
Handles fundamental program operations, account management, and state transitions. This layer implements the core business logic of the program.
Security Layer
Implements access controls, signature verification, and security validations to ensure only authorized operations can be performed.
Integration Layer
Manages interactions with external programs and services, including Metaplex, Token Program, and price oracles through Cross-Program Invocations (CPIs).
Data Layer
Defines the account structures and data models used throughout the program, ensuring efficient storage and retrieval of on-chain data.
Instruction Layer
Processes incoming instructions, validates parameters, and routes operations to the appropriate handlers in the core layer.
Utility Layer
Provides helper functions, utilities, and common operations used across the program to reduce code duplication and ensure consistency.
The program uses a structured data model to store and manage NFT collections, staking pools, and user interactions. All data is stored on-chain using Solana's account model.
#[account]
pub struct StakingPool {
pub reward_rate: u64, // Rewards per day
pub staked_nfts: Vec<Pubkey>,
pub total_staked: u64,
pub last_update: i64,
}
#[account]
pub struct Collection {
pub name: String,
pub symbol: String,
pub creator: Pubkey,
pub royalty_basis_points: u16,
}
The program exposes a set of instructions that clients can invoke to interact with the system. Each instruction is handled by a dedicated function that implements the required logic.
pub fn mint_nft(ctx: Context<MintNft>, uri: String, title: String) -> Result<()>
pub fn initialize_staking_pool(ctx: Context<InitializeStakingPool>, reward_rate: u64) -> Result<()>
pub fn stake_nft(ctx: Context<StakeNft>, nft: Pubkey) -> Result<()>
pub fn collect_fees(ctx: Context<CollectFees>, amount: u64) -> Result<()>
pub fn send_nft(ctx: Context<SendReceive>, nft: Pubkey, recipient: Pubkey) -> Result<()>
Solana Token Program
Used for creating and managing tokens, including minting, transferring, and burning.
Metaplex Token Metadata
Handles NFT metadata, including creation, updates, and verification.
System Program
Core Solana program for creating accounts and transferring SOL.
Pyth Price Oracle
Provides real-time price data for tokens and assets.
The Gold Digger Factory Program implements several security measures to protect user assets and ensure the integrity of operations:
- Rigorous signature verification for all transactions
- Program Derived Addresses (PDAs) for secure account management
- Comprehensive input validation to prevent exploits
- Access control checks for privileged operations
- Secure handling of token transfers and NFT operations
- Protection against reentrancy attacks